Objective
- Understand how to use the gpio driver library from
Espressif
. The lab will consist of using a sweeper
, led chaser
and any additional led sequence that the student developed and be selected via an input. Students must have a total of three buttons. First button must start sweeper
function which student must have completed from the previous lab. Next, the second button must start the led chaser
function. Lastly, the third button must toggle the state of the onboard led.
Button | Function | Mode |
B0 | Sweeper | Pull-down |
B1 | Led chaser | Pull-down |
B2 | Toggle | Pull-down |
Bonus
- Undergrad Bonus:
- Create another
LED
sequence and add another button, as pull-up
.
- Grad Bonus:
- Add button to start lightshow from previous lab, as
pull-up
- Do a reset button which will turns off all LEDs, as
pull-up
ESP32 Pinout
+-----------------------+
| O | USB | O |
| ------- |
3V3 | [ ] [ ] | VIN
GND | [ ] [ ] | GND
Touch3 / HSPI_CS0 / ADC2_3 / GPIO15 | [ ] [ ] | GPIO13 / ADC2_4 / HSPI_ID / Touch4
CS / Touch2 / HSPI_WP / ADC2_2 / GPIO2 | [ ] [ ] | GPIO12 / ADC2_5 / HSPI_Q / Touch5
Touch0 / HSPI_HD / ADC2_0 / GPIO4 | [ ] [ ] | GPIO14 / ADC2_6 / HSPI_CLK / Touch6
U2_RXD / GPIO16 | [ ] [ ] | GPIO27 / ADC2_7 / Touch7
U2_TXD / GPIO17 | [ ] [ ] | GPIO26 / ADC2_9 / DAC2
V_SPI_CS0 / GPIO5 | [ ] ___________ [ ] | GPIO25 / ADC2_8 / DAC1
SCK / V_SPI_CLK / GPIO18 | [ ] | | [ ] | GPIO33 / ADC1_5 / Touch8 / XTAL32
U0_CTS / MSIO / V_SPI_Q / GPIO19 | [ ] | | [ ] | GPIO32 / ADC1_4 / Touch9 / XTAL32
SDA / V_SPI_HD / GPIO21 | [ ] | | [ ] | GPIO35 / ADC1_7
CLK2 / U0_RXD / GPIO3 | [ ] | | [ ] | GPIO34 / ADC1_6
CLK3 / U0_TXD / GPIO1 | [ ] | | [ ] | GPIO39 / ADC1_3 / SensVN
SCL / U0_RTS / V_SPI_WP / GPIO22 | [ ] | | [ ] | GPIO36 / ADC1_0 / SensVP
MOSI / V_SPI_WP / GPIO23 | [ ] |___________| [ ] | EN
| |
| | | ____ ____ | |
| | | | | | | | |
| |__|__| |__| |__| |
| O O |
+-----------------------+
Example
Here is an example of how to use ESP32 GPIO function calls for inputs. The following code will turn the onboard LED as long as the button is pressed.
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "driver/gpio.h"
#define LOW 0
#define HIGH 1
#define ONBOARD_LED 2
#define BUTTON_0 23
esp_rom_gpio_pad_select_gpio(BUTTON_0);
gpio_set_direction(BUTTON_0, GPIO_MODE_INPUT);
gpio_set_pull_mode(BUTTON_0, GPIO_PULLDOWN_ONLY);
while(1){
int res = gpio_get_level(BUTTON_0);
vTaskDelay(10/portTICK_PERIOD_MS);
if(res == 1){
}else{
}
vTaskDelay(100/portTICK_PERIOD_MS);
}
}
void app_main()
Definition main.c:261
#define HIGH
Definition main.c:28
#define LOW
Definition main.c:27
#define ONBOARD_LED
Definition main.c:31
Lab Template
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "driver/gpio.h"
#define LOW 0
#define HIGH 1
{
for (int i = 0; i < size; i++)
{
}
return;
}
{
for (int i = 0; i < size; i++)
{
}
return;
}
void sweep(uint8_t *led,
int size)
{
}
}
{
}
{
uint8_t led[] = {13};
uint8_t button[] = {22, 23};
int led_size = sizeof(led) / sizeof(uint8_t);
int button_size = sizeof(button) / sizeof(uint8_t);
while (1)
{
vTaskDelay(5/portTICK_PERIOD_MS);
int button0 = gpio_get_level(button[0]);
if(button0 == 1){
}
else{
vTaskDelay(100/portTICK_PERIOD_MS);
}
}
}
void setOutputs(uint8_t *out, int size)
setOutputs will initialize uint8_t array as outputs
Definition main.c:38
void led_chaser(uint8_t *led, int size)
led_chaser led chaser will make a single led to iterate over the array
Definition main.c:77
void sweep(uint8_t *led, int size)
sweep function will sweep among the GPIOs
Definition main.c:56
void light_show()
Definition main.c:92
void setInputs(uint8_t *in, int size)
setInputs will initialize uint8_t array as inputs
Definition main.c:47
C helpful functions
For this lab, there are additional functions from Espressif that are important for using inputs. As the previous lab, we must set the direction of the GPIO pin by using gpio_set_direction(gpio_num_t gpio_num, gpio_mode_t mode)
. Previously we used GPIO_MODE_OUTPUT
as we using outputs, however, now that we will be using inputs, we need GPIO_MODE_INPUT
.
esp_err_t gpio_set_direction(gpio_num_t gpio_num, gpio_mode_t mode);
Next, as the name states gpio_get_level(gpio_num_tgpio_num)
returns the current level of the GPIO pin whether it high and low. This function is use to get the current state of the input.
int gpio_get_level(gpio_num_ tgpio_num);
Lastly, there are two additional functions that are crucial when using inputs. There are two configurations for inputs either pull-up or pull-down: gpio_pulldown_en(gpio_num_t gpio_num)
and gpio_pullup_en(gpio_num_t gpio_num)
. Down below are the configuration for both pull-up and pull-down.
esp_err_t gpio_pullup_en(gpio_num_t gpio_num);
esp_err_t gpio_pulldown_en(gpio_num_t gpio_num);
Pull-up and Pull-down Configuration
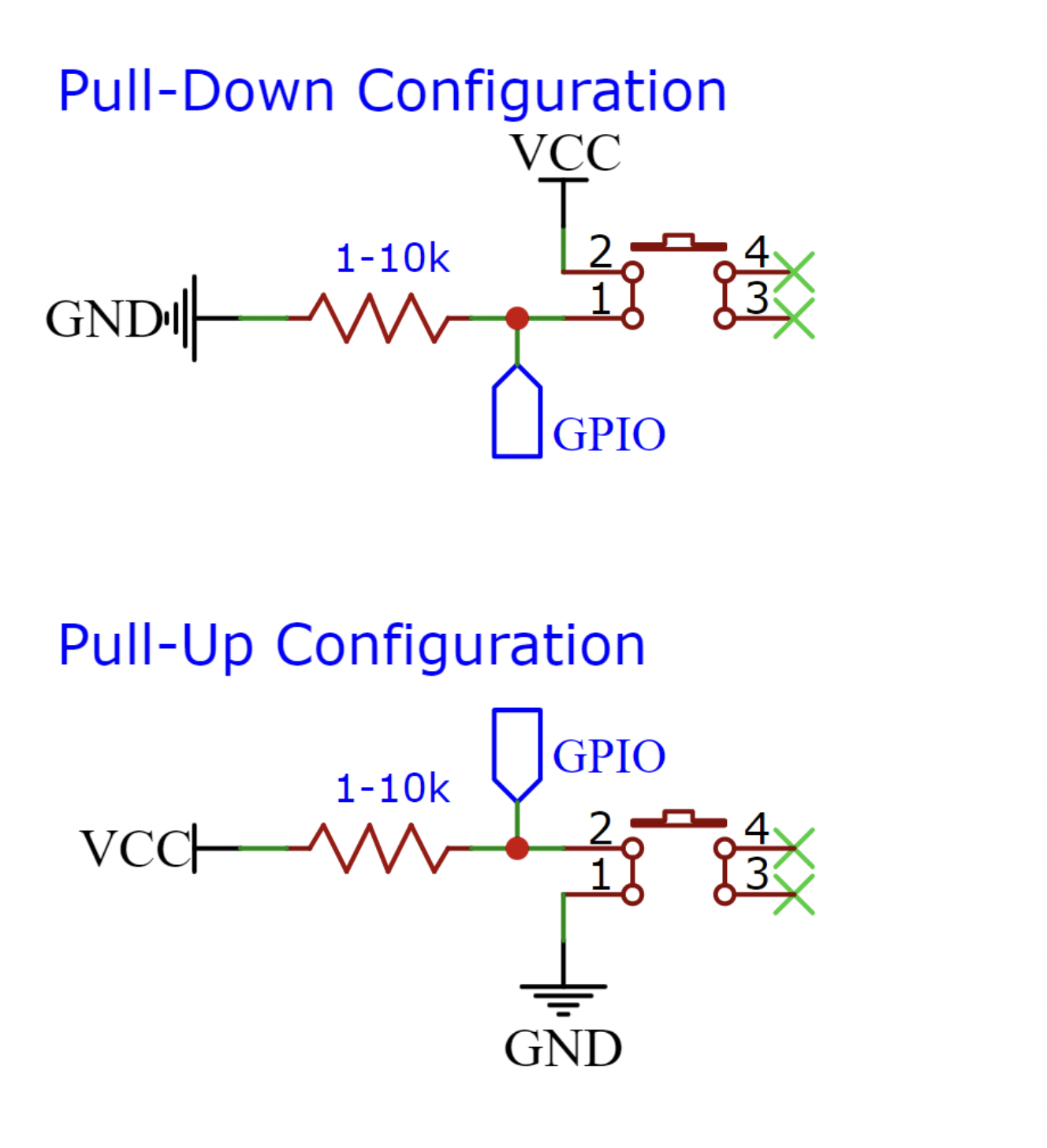
Additional Links
Authors
GitHub
Read Next: Lab 2